Lesson 14: PHP Array Functions
Have a Question?
Latest Articles
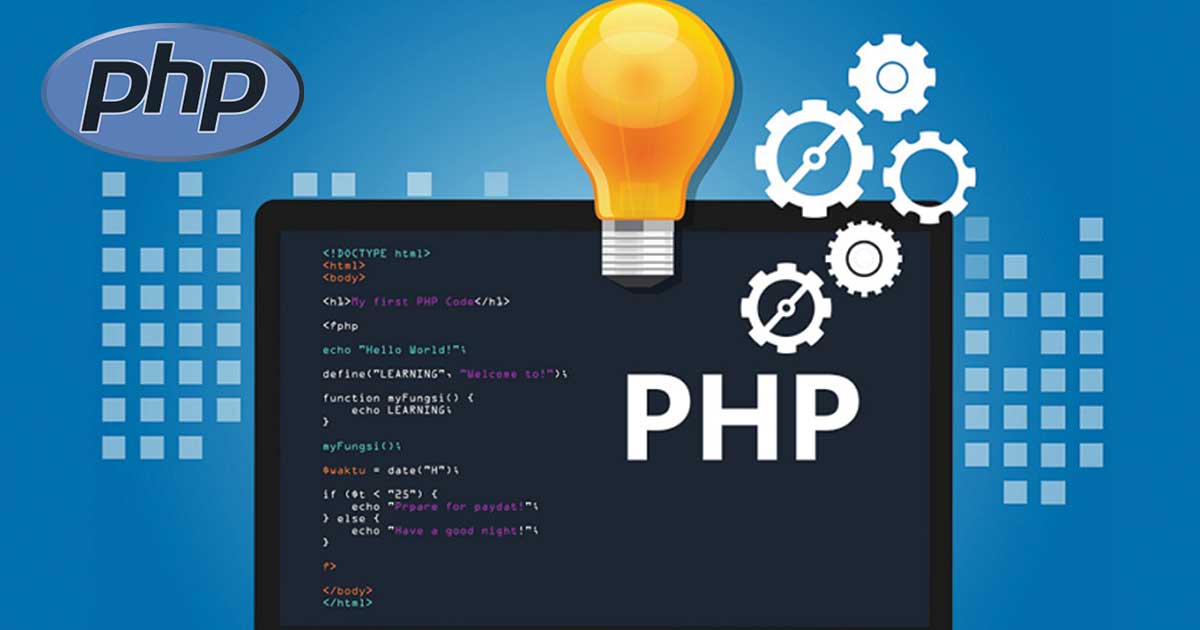
Lesson 14: PHP Array Functions
Arrays are one of the most commonly used data structures in PHP. Thankfully, PHP offers a wide range of built-in functions that simplify array handling. In this article, you’ll discover the most useful array functions, categorized by their purpose, with clear and concise examples.
1. Functions to Add Elements
array_push()
Adds one or more elements to the end of an array.
$arr = ['PHP', 'JavaScript'];
array_push($arr, 'Python');
print_r($arr);
array_unshift()
Adds an element to the beginning of an array.
$arr = ['PHP', 'JavaScript'];
array_unshift($arr, 'HTML');
print_r($arr);
2. Search Functions
in_array()
Checks if a value exists in an array.
$skills = ['HTML', 'CSS', 'PHP'];
if (in_array('PHP', $skills)) {
echo "Found!";
}
array_search()
Searches for a value and returns its key.
$skills = ['frontend' => 'HTML', 'backend' => 'PHP'];
$key = array_search('PHP', $skills);
echo $key;
3. Removing Elements
array_pop()
Removes the last element of an array.
$stack = ['first', 'second', 'third'];
array_pop($stack);
print_r($stack);
array_shift()
Removes the first element.
$queue = ['start', 'middle', 'end'];
array_shift($queue);
print_r($queue);
4. Sorting Functions
sort()
Sorts elements in ascending order.
$numbers = [5, 3, 9, 1];
sort($numbers);
print_r($numbers);
rsort()
Sorts in descending order.
$letters = ['b', 'a', 'd', 'c'];
rsort($letters);
print_r($letters);
5. Filtering and Mapping
array_filter()
Filters elements based on a condition.
$nums = [1, 2, 3, 4, 5];
$even = array_filter($nums, fn($n) => $n % 2 === 0);
print_r($even);
array_map()
Applies a function to each array item.
$nums = [1, 2, 3];
$squares = array_map(fn($n) => $n * $n, $nums);
print_r($squares);
Conclusion
PHP array functions save time and improve efficiency. By mastering them, developers can handle data processing tasks more effectively. Try applying them in real-world projects for better results.
Important links Portfolio
Share with your friends