Lesson 11: PHP Loops (while - do...while - for - foreach)
Have a Question?
Latest Articles
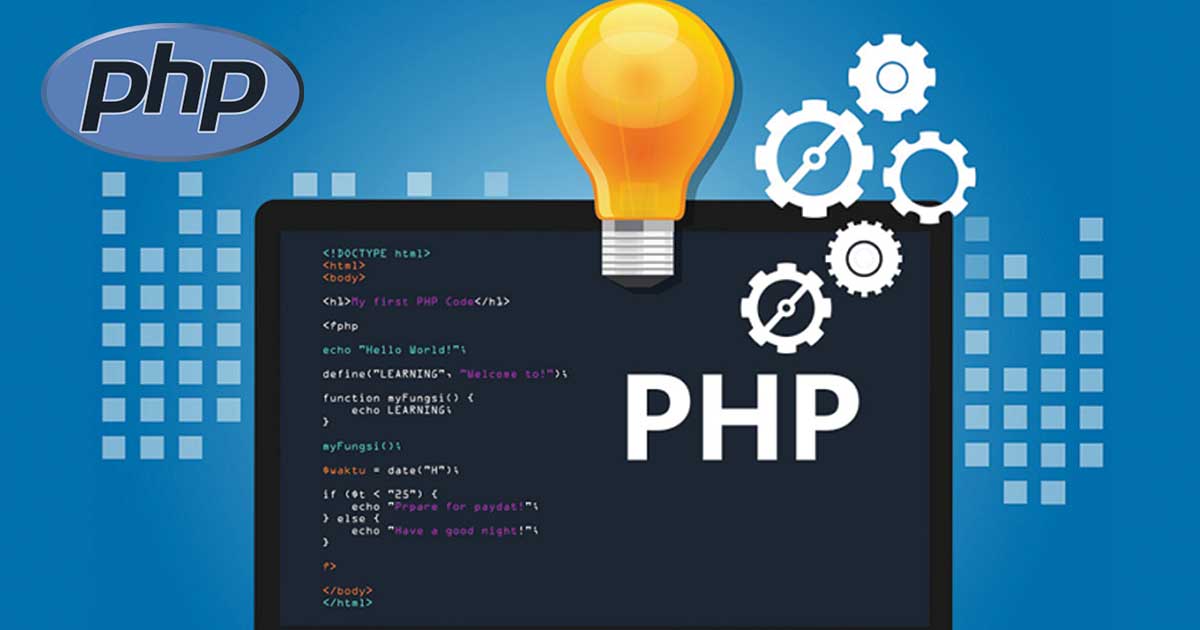
Lesson 11: PHP Loops (while - do...while - for - foreach)
Loops are one of the most important tools in programming. They allow us to repeat blocks of code based on specific conditions. In PHP, there are four main types of loops: while
, do...while
, for
, and foreach
. This article will explore each type with practical examples.
The while Loop
The while
loop continues to execute the block of code as long as the condition is true.
$x = 1;
while ($x <= 5) {
echo "The number is: $x <br>";
$x++;
}
When to use it?
When the number of repetitions is unknown in advance.
The do...while Loop
This loop is similar to while
, but it guarantees at least one execution, even if the condition is false.
$x = 1;
do {
echo "The number is: $x <br>";
$x++;
} while ($x <= 5)
Key Difference
do...while
executes first, then checks the condition.while
checks the condition before execution.
The for Loop
Used when the number of repetitions is known. It includes initialization, condition, and increment.
for ($i = 1; $i <= 5; $i++) {
echo "The number is: $i <br>";
}
Advantages
Perfect for fixed counts.
Easy to understand and manage.
The foreach Loop
Designed specifically for arrays. It iterates through each element without manual indexing.
$fruits = ["Apple", "Banana", "Orange"];
foreach ($fruits as $fruit) {
echo "Fruit: $fruit <br>";
}
When to use it?
When looping through arrays in a simple and clean way.
Conclusion
Each PHP loop serves a specific purpose. Choosing the right loop enhances code readability and performance. Practice using them to understand their unique behaviors.
Important links Portfolio
Share with your friends