Lesson 10: PHP switch Statement
Have a Question?
Latest Articles
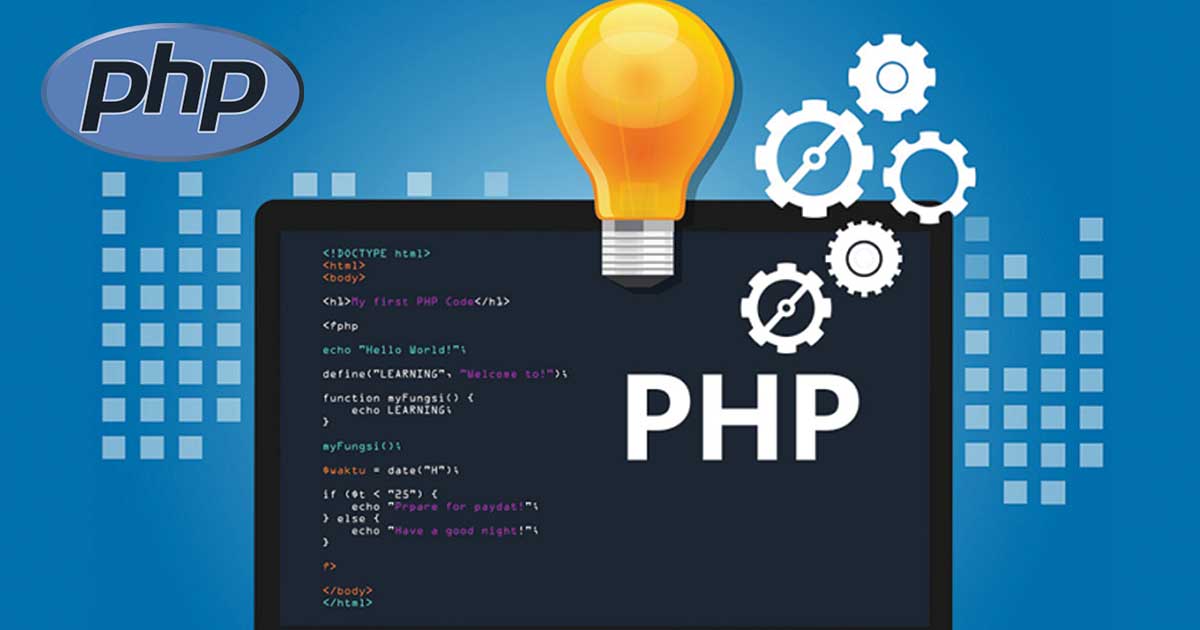
Lesson 10: PHP switch Statement
What is the switch statement in PHP?
The switch
statement in PHP is used to execute different blocks of code based on the value of a single variable. It's a cleaner alternative to writing multiple if...else
statements.
When to use switch?
Use the switch
statement when you have one variable and want to compare it against several possible values. This approach makes the code easier to read and manage.
Syntax of switch in PHP
switch ($variable) {
case 'value1':
// Code executed if value is value1
break;
case 'value2':
// Code executed if value is value2
break;
default:
// Code executed if no case matches
}
Components of the switch statement
switch($variable): Evaluates the variable's value.
case 'value': Executes the code if the value matches.
break: Prevents code from falling through to the next case.
default: Executes if no cases match.
Practical example of switch
$day = "Tuesday";
switch ($day) {
case "Monday":
echo "Today is the start of the week.";
break;
case "Tuesday":
echo "Today is the second day of the week.";
break;
default:
echo "Today is neither Monday nor Tuesday.";
}
Output:
Today is the second day of the week.
Benefits of using switch
Makes the code more organized and readable.
Improves performance when checking many conditions.
Useful when dealing with fixed sets of values.
Tips for writing effective switch statements
Always use
break
to prevent accidental fall-through.Include a
default
case for unexpected values.Keep the code clean and well-commented when necessary.
Conclusion
The switch
statement in PHP is a powerful and readable way to handle multiple conditions. It simplifies complex if...else
structures and improves the logic flow in your application. Use it wisely to write better code.
Important links Portfolio
Share with your friends