Lesson 9: PHP if...elseif...else
Have a Question?
Latest Articles
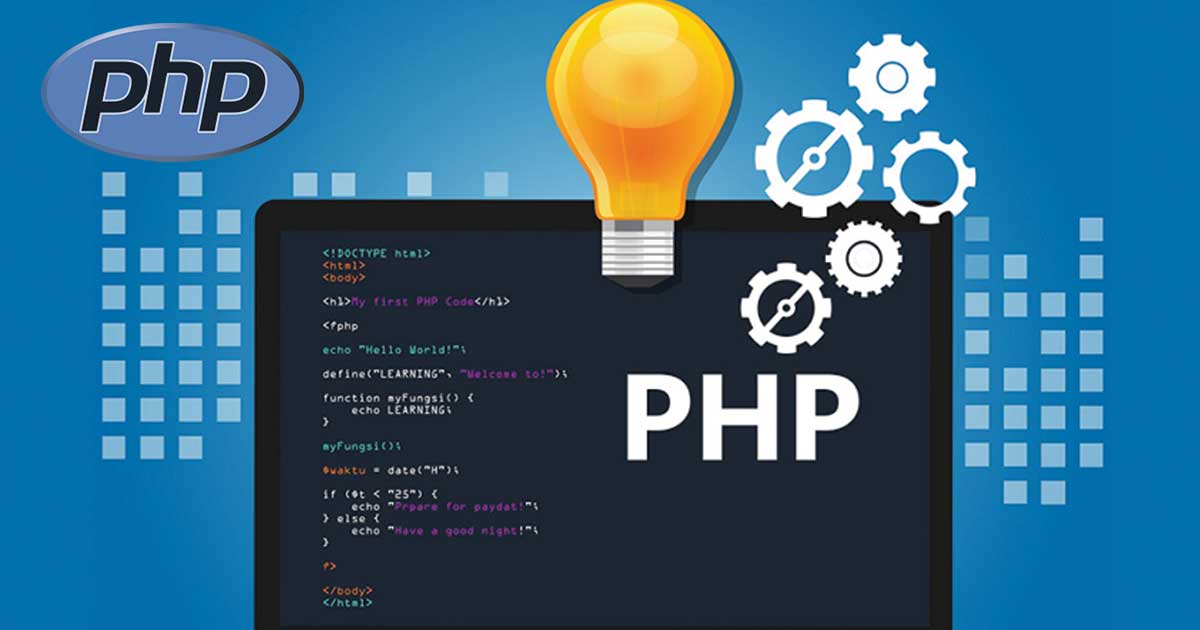
Lesson 9: PHP if...elseif...else
In PHP programming, conditional statements are essential building blocks. Among them, the if...elseif...else
structure allows developers to make decisions based on different conditions.
In this article, we will explore how to use this structure effectively, with simple examples suitable for beginners and experienced developers alike.
What is the if
statement in PHP?
The if
statement is used to execute a block of code only if a specific condition is true.
Basic syntax:
if (condition) { // code to execute if condition is true }
Example:
$age = 18;
if ($age >= 18) {
echo "You are an adult.";
}
Using elseif
to handle multiple conditions
When you want to check several conditions, use elseif
, which means "else if".
Example:
$grade = 85;
if ($grade >= 90) {
echo "Excellent";
} elseif ($grade >= 75) {
echo "Very Good";
} elseif ($grade >= 60) {
echo "Good";
}
When to use else
Use the else
statement when none of the previous conditions are true. It acts as a fallback.
Example:
$score = 45;
if ($score >= 90) {
echo "Excellent";
} elseif ($score >= 75) {
echo "Very Good";
} else {
echo "Needs Improvement";
}
Important Tips
Always order conditions from most specific to least specific.
Use curly braces
{}
to clarify the code structure.Keep conditions short and meaningful.
Conclusion
The if...elseif...else
statements are fundamental in PHP development. They allow you to control the flow of your code and make logical decisions.
Try writing your own examples and experiment with different values to see how they work in action.
Important links Portfolio
Share with your friends