Lesson 2: PHP Syntax
Have a Question?
Latest Articles
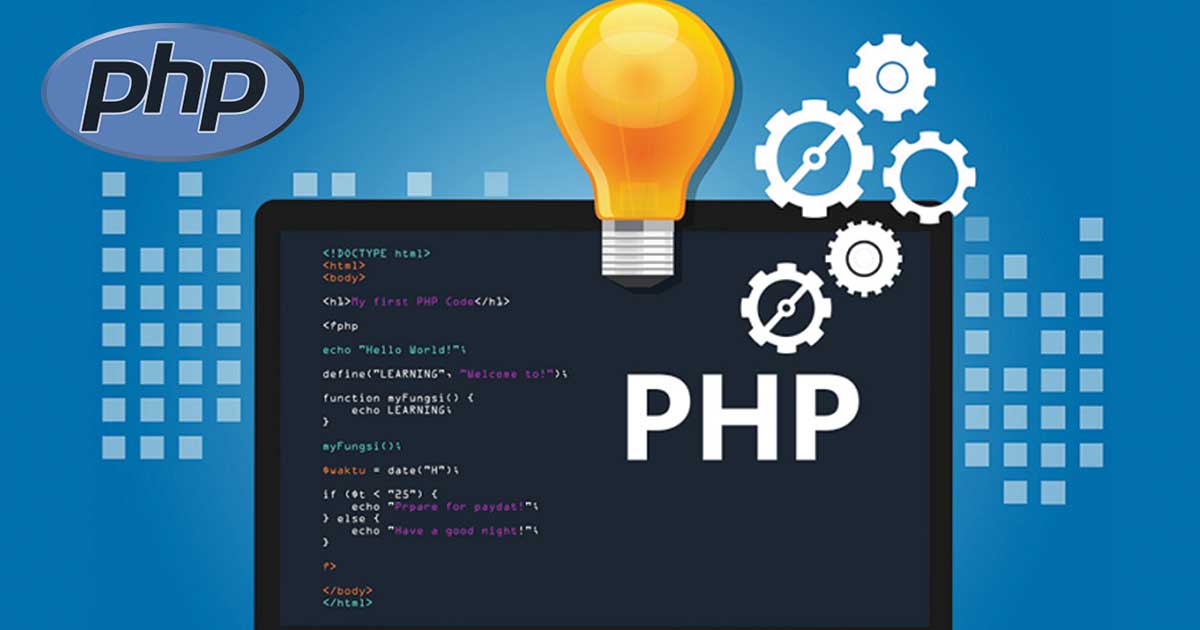
Lesson 2: PHP Syntax
PHP is one of the most popular programming languages for web development. It features a simple syntax and offers great flexibility. In this article, we’ll explore the basics of PHP syntax and how to write clean, readable code.
1. How to Start a PHP Code Block
Every PHP script must begin with:
<?php
// your code
?>
You can omit the closing tag ?>
in pure PHP files. Doing so helps avoid unexpected output issues.
2. Comments in PHP
Comments make your code easier to understand. PHP ignores them during execution.
Single-line comment:
// This is a comment
Multi-line comment:
/* This is a multi-line comment */
3. Variables
Variables in PHP start with a $
symbol followed by the name:
$name = "Alaa";
$age = 30;
Rules:
Must start with a letter or underscore
Cannot start with a number
Are case-sensitive
4. Data Types in PHP
Common data types include:
String
Integer
Float
Boolean
Array
Object
NULL
5. Conditional Statements
Use conditions to make decisions:
if ($age > 18) {
echo "You are an adult.";
} else {
echo "You are a minor.";
}
6. Loops in PHP
Loops allow you to repeat actions:
for ($i = 0; $i < 5; $i++) {
echo $i;
}
7. Functions
A function is a reusable block of code:
function sayHello($name) {
return "Hello, " . $name;
}
To call the function:
echo sayHello("Alaa");
Conclusion
Mastering PHP syntax is the first step to becoming a skilled web developer. Practice writing code and experiment with different features. The more you code, the better you'll get.
Important links Portfolio
Share with your friends